Working with Arrays in Javascript
Introduction to Arrays in Javascript
Arrays are an important data structure in Javascript. They allow you to store multiple values in a single variable. In Javascript, arrays can hold different types of data, such as numbers, strings, or even other arrays.
Common Array Operations and Functions
Once you have an array, you can perform various operations on it. Some common array operations include:
- Accessing array elements using their index
- Adding elements to an array
- Removing elements from an array
- Iterating over an array
- Sorting and searching arrays
Javascript provides built-in array functions, such as push
, pop
, shift
, and unshift
, to add or remove elements from an array. You can also use the length
property to get the number of elements in an array, and the forEach
method to iterate over each element.
Here’s an example of how to add and remove elements from an array using the built-in functions:
let fruits = ["apple", "banana", "orange"];console.log(fruits); // Output: ["apple", "banana", "orange"]fruits.push("grape");console.log(fruits); // Output: ["apple", "banana", "orange", "grape"]fruits.pop();console.log(fruits); // Output: ["apple", "banana", "orange"]
Advanced Array Manipulation Techniques
Besides the common array operations, there are advanced techniques that can help you manipulate arrays more efficiently. Some of these techniques include:
- Using
map
,filter
, andreduce
to transform and aggregate array elements - Using
splice
to insert, replace, or remove elements at specific positions - Using
slice
to extract a portion of an array - Using
concat
to combine multiple arrays
Let’s see an example of using the map
function to transform an array of numbers:
let numbers = [1, 2, 3, 4, 5];let doubledNumbers = numbers.map(function(num) { return num * 2;});console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
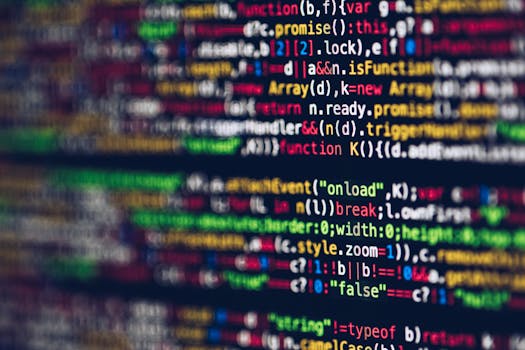
These advanced techniques can greatly simplify your code and make it more readable and maintainable.
In conclusion, arrays are a fundamental concept in Javascript programming. Understanding how to work with arrays, including common operations and advanced techniques, will empower you to write more efficient and powerful Javascript code.